No.54244 [Last50 Posts]
it's been a long time since I've made a new thread~!
happy December, everyone~!
____________________________
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54245
>>54242
yeah, I don't need to save any data, only take the data given to me, run calculations, then display via labels
>>54243
okay, it isn't until sunday, after all.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54246
>>54242
he posted an image
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54247
Here is what I think would make Poppy's code work.
If you need to make all of the subprocedures work together, you need to define the variables which they are meant to use in a place which is visible to all of the procedures.
/* ========================================================================== */
/* */
/* Filename.c */
/* (c) 2001 Author */
/* */
/* Description */
/* */
/* ========================================================================== */
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Smoothie_Queen_Calculator___Final_Project
{
public partial class Form1 : Form
{
decimal subtotal;
decimal extras;
decimal discount;
decimal tax;
decimal total;
decimal[,] smoothieListDecimal = {{ 5.99M, 6.99M, 7.99M, 8.99M },
{ 6.99M, 7.99M, 8.99M, 9.99M }};
int quantityval;
float quantity;
public Form1()
{
InitializeComponent();
total = 0m;
}
private void calculatebtn_Click(object sender, EventArgs e)
{
string customername = customernametxt.Text;
if (customername.Length == 0)
{
MessageBox.Show("Please enter the customer name");
return;
}
bool tempval = Single.TryParse(quantitytxt.Text, out quantity);
if (!tempval)
{
MessageBox.Show("Please enter a numeric quantity");
return;
}
CalculateSubTotal();
CalculateExtras();
CalculateDiscount();
AddTax();
Finalize();
}
private void CalculateSubTotal()
{
if (sizecb.SelectedIndex != -1 && stylecb.SelectedIndex != -1)
{
subtotal = smoothieListDecimal[stylecb.SelectedIndex, sizecb.SelectedIndex];
}
else
{
MessageBox.Show("Please select size and style.");
return;
}
subtotal = subtotal * quantityval;
total += subtotal;
}
private void CalculateExtras()
{
if (echinaceacb.Checked)
{
extras += 0.75m;
}
if (beepollencb.Checked)
{
extras += 0.75m;
}
if (energyboostercb.Checked)
{
extras += 0.75m;
}
extras = extras * quantityval;
total += extras;
}
private void CalculateDiscount()
{
decimal discount = 0m;
decimal total = 0;
if (couponrb.Checked)
{
discount = total* 0.1m;
}
else if(preferredrb.Checked)
{
discount = total* 0.15m;
}
total -= discount;
}
private void AddTax()
{
tax = total* 0.08m;
total += tax;
}
private void Finalize()
{
subtotallbl.Text = subtotal.ToString("C");
extraslbl.Text = extras.ToString("C");
discountlbl.Text = discount.ToString("C");
salestaxlbl.Text = tax.ToString("C");
amountduelbl = total.ToString("C");
}
private void exitbtn_Click(object sender, EventArgs e)
{
this.Close();
}
private void clearbtn_Click(object sender, EventArgs e)
{
}
private void aboutbtn_Click(object sender, EventArgs e)
{
}
private void exitToolStripMenuItem_Click(object sender, EventArgs e)
{
this.Close();
}
}
}
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54248
oh, I had them in a void, I'm silly, I was just doing it in notepad so I didn't notice, whoopsss
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54249
>>54246
I know.
>>54245
>yeah, I don't need to save any data, only take the data given to me, run calculations, then display via labels
I guess you are right, at least the assignment doesn't seem to mention that you should be able to click it multiple times and get the sum for all of the suborders.
>>54248
It happens ^.^
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54250
>>54249
>I guess you are right
In which case, you should indeed put
total = 0m;
back at the beginning of private void calculatebtn_Click(object sender, EventArgs e)
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54251
>>54249
i-if you know, why'd you ask?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54252
>>54251
I was asking you how you make the code coloured on 8chan, like you did in >>54210
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54253
>>54247
it doesn't like this for some reason
also it is doing something, but it still isn't displaying the error box if a style and size isn't picked, and it isn't doing any of the math
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54254
>>54250
I don't really think three people need to be editing the same code
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Smoothie_Queen_Calculator___Final_Project
{
public partial class Form1 : Form
{
string customername = customernametxt.Text;
int quantityval;
decimal subtotal;
decimal extras;
decimal discount;
decimal tax;
decimal total;
decimal[,] smoothieListDecimal = {{ 5.99M, 6.99M, 7.99M, 8.99M },
{ 6.99M, 7.99M, 8.99M, 9.99M }};
public Form1()
{
InitializeComponent();
}
private void calculatebtn_Click(object sender, EventArgs e)
{
if (customername.Length == 0)
{
MessageBox.Show("Please enter the customer name.");
return;
}
bool tempval = Int32.TryParse(quantity.Text, out quantityval);
if (!tempval)
{
MessageBox.Show("Please enter a numeric quantity.");
return;
}
if(quantityval == 0)
{
MessageBox.Show("Quantity must not be zero.");
return;
}
total = 0m;
CalculateSubTotal();
CalculateExtras();
CalculateDiscount();
AddTax();
Finalize();
}
private void CalculateSubTotal();
{
if (sizecb.SelectedIndex != -1 && stylecb.SelectedIndex != -1)
{
subtotal = smoothieListDecimal[stylecb.SelectedIndex, sizecb.SelectedIndex];
}
else
{
MessageBox.Show("Please select size and style.");
return;
}
subtotal = subtotal * quantityval;
total += subtotal;
}
private void CalculateExtras();
{
extras = 0m;
if(echinaceacb.Checked)
{
extras += 0.75m;
}
if(beepollencb.Checked)
{
extras += 0.75m;
}
if(energyboostercb.Checked)
{
extras += 0.75m;
}
extras = extras * quantityval;
total += extras;
}
private void CalculateDiscount();
{
discount = 0m;
if(discountCoupon.Checked)
{
discount = total * 0.1m;
}
else if(discountPreferred.Checked)
{
discount = total * 0.15m;
}
total -= discount;
}
private void AddTax();
{
tax = total * 0.08m;
total += tax;
}
private void Finalize();
{
subtotallbl.Text = subtotal.ToString("C");
extraslbl.Text = extras.ToString("C");
discountlbl.Text = discount.ToString("C");
salestaxlbl.Text = tax.ToString("C");
amountduelbl = total.ToString("C");
}
}
}
does this work?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54255
>>54253
you forgot to put '.Text' after "amountduelbl"
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54256
>>54253
Huh, that is weird.
If you compile it, does it give you any error message?
Oh never mind Poppy got it X3
>>54254
I was just trying to sum up all the changes I was suggesting into one post.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54257
>>54256
no changes are necessary
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54258
it's just getting confusing with three different versions of the same code, is what I meant, s-sorry
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54259
>>54255
thank you
>>54256
>>54258
okay, so we'll just all work with poppy's code
>>54254
it doesn't like when you put ";" at the end of the voids
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54260
>>54259
oh sorry, those don't go there, editing without colors or formatting is bullying me
once you remove those, does it give any errors?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54261
>>54257
Yes they were, the definition of variables in the right place were necessary!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54262
>>54254
dont put ; when defining functions, only when calling them
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54263
>>54260
yeah a few, i can't copy paste your code due to the buttons, so I am trying to get it as close as possible
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54264
>>54263
>i can't copy paste your code due to the buttons
Those have different names, yeah? So if you just find&replace them with the right names, you should be fine, yes?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54265
what I am working with now
the errors all come up, but still no math being displayed
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Smoothie_Queen_Calculator___Final_Project
{
public partial class Form1 : Form
{
string customername = customernametxt.Text;
int quantityval;
decimal subtotal;
decimal extras;
decimal discount;
decimal tax;
decimal total;
decimal[,] smoothieListDecimal = {{ 5.99M, 6.99M, 7.99M, 8.99M },
{ 6.99M, 7.99M, 8.99M, 9.99M }};
public Form1()
{
InitializeComponent();
}
private void calculatebtn_Click(object sender, EventArgs e)
{
if (customername.Length == 0)
{
MessageBox.Show("Please enter the customer name");
return;
}
bool tempval = Single.TryParse(quantitytxt.Text, out quantity);
if (!tempval)
{
MessageBox.Show("Please enter a numeric quantity");
return;
}
total = 0m;
CalculateSubTotal();
CalculateExtras();
CalculateDiscount();
AddTax();
Finalize();
}
private void CalculateSubTotal()
{
if (sizecb.SelectedIndex != -1 && stylecb.SelectedIndex != -1)
{
subtotal = smoothieListDecimal[stylecb.SelectedIndex, sizecb.SelectedIndex];
}
else
{
MessageBox.Show("Please select size and style.");
return;
}
subtotal = subtotal * quantityval;
total += subtotal;
}
private void CalculateExtras()
{
if (echinaceacb.Checked)
{
extras += 0.75m;
}
if (beepollencb.Checked)
{
extras += 0.75m;
}
if (energyboostercb.Checked)
{
extras += 0.75m;
}
extras = extras * quantityval;
total += extras;
}
private void CalculateDiscount()
{
if (couponrb.Checked)
{
discount = total* 0.1m;
}
else if(preferredrb.Checked)
{
discount = total* 0.15m;
}
total -= discount;
}
private void AddTax()
{
tax = total* 0.08m;
total += tax;
}
private void Finalize()
{
subtotallbl.Text = subtotal.ToString("C");
extraslbl.Text = extras.ToString("C");
discountlbl.Text = discount.ToString("C");
salestaxlbl.Text = tax.ToString("C");
amountduelbl = total.ToString("C");
}
private void exitbtn_Click(object sender, EventArgs e)
{
this.Close();
}
private void clearbtn_Click(object sender, EventArgs e)
{
}
private void aboutbtn_Click(object sender, EventArgs e)
{
}
private void exitToolStripMenuItem_Click(object sender, EventArgs e)
{
this.Close();
}
}
}
>>54264
it makes it freak out, tho
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54266
>>54265
which lines the errors at?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54267
>>54265
you forgot .Text again after the one amountlbl
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54268
>>54265
don't call it Finalized, rename it
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54269
>>54266
here and here
>>54267
fixed that
>>54268
okay
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54270
>>54269
out quantityval, not quantity
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54271
>>54269
the line numbers of the errors?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54272
>>54270
fixed, but now it is giving me an error
>>54271
here are all the lines of code with numbers
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54273
>>54272
change Single.TryParse to Int32.TryParse
change "finished" to "UpdateValues()"
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54274
>>54269
Finalize was the one that was reserved, not Finalized. just call it displayAll or something
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54275
>>54272
do none of these have errors?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54276
>>54272
updatevalues seems like maybe it's used too, just call it something random, like holo anon's "displayAll" or "PushValues" or whatever you want
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54277
>>54276
okay
>>54275
it isn't giving me any
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54278
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54279
>>54272
>fixed, but now it is giving me an error
string customername = customernametxt.Text;
So, is customernametxt your object, or is it copypaste from Poppy?
>>54272
>>54275
Also there might be an error in assigning that value ↑ there instead of in the constructor thing, no? Is it valid to assign a non-static value in the class definition?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54280
>>54279
>non-static value
Or maybe I meant *non-constant.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54281
>>54279
no, it is the name of the textbox
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54282
don't forget to set the discount to 0m at the start of the discount calculation
private void CalculateDiscount()
{
discount = 0m;
if(couponrb.Checked)
it won't error but it might cause issues later if we forget
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54283
>>54279
it's logically wrong; you want the value of the text at the time the buy button is clicked
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54284
>>54279
>>54283
thanks for pointing that out
private void calculatebtn_Click(object sender, EventArgs e)
{
string customername = customernametxt.Text;
silly dumb poppy
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54285
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54286
>>54281
Okays. Then do what anon and Poppy say and move that assignment into the clickey subprocedure.
>>54283
>>54284
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54287
>>54285
put it at the start of the CalculateDiscount void, I don't know how visual C# works but we're adding to the value and never removing from it otherwise, so it won't go to 0 if there's no coupon, if there was one before, possibly
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54288
>>54284
string customerName;
private void calc.(...) {
customerName = customernametxt.Text;
...
}
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54289
>>54286
>>54287
holy shit
it fucking works guys
you were right tho mouse
it does stack, and it thinks the extras, are more then they are, but it fucking works
woooooooo
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54290
there is also something off about the discount, but it fucking works
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54291
>>54289
Yay, progress!
>it does stack
What stacks? o:
Post your current code please.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54292
>>54289
when you test, you test the error cases also
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54293
>>54289
yay~! I'm glad that you won't have to pay someone monster-anon, that wouldn't be good at all
>>54290
would you be able to post the entire code again?
also, add this to the last function, updatevalues or whatever you called it
subtotallbl.Text = subtotal.ToString("C");
extraslbl.Text = extras.ToString("C");
discountlbl.Text = discount.ToString("C");
salestaxlbl.Text = tax.ToString("C");
amountduelbl.Text = total.ToString("C");
subtotal = 0m;
extras = 0m;
discount = 0m;
tax = 0m;
total = 0m;
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54294
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54295
>>54291
>>54293
sure thing
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Smoothie_Queen_Calculator___Final_Project
{
public partial class Form1 : Form
{
int quantityval;
decimal subtotal;
decimal extras;
decimal discount = 0M;
decimal tax;
decimal total;
decimal[,] smoothieListDecimal = {{ 5.99M, 6.99M, 7.99M, 8.99M },
{ 6.99M, 7.99M, 8.99M, 9.99M }};
public Form1()
{
InitializeComponent();
}
private void calculatebtn_Click(object sender, EventArgs e)
{
string customername = customernametxt.Text;
if (customername.Length == 0)
{
MessageBox.Show("Please enter the customer name");
return;
}
bool tempval = Int32.TryParse(quantitytxt.Text, out quantityval);
if (!tempval)
{
MessageBox.Show("Please enter a numeric quantity");
return;
}
total = 0m;
CalculateSubTotal();
CalculateExtras();
CalculateDiscount();
AddTax();
displayAll();
}
private void CalculateSubTotal()
{
if (sizecb.SelectedIndex != -1 && stylecb.SelectedIndex != -1)
{
subtotal = smoothieListDecimal[stylecb.SelectedIndex, sizecb.SelectedIndex];
}
else
{
MessageBox.Show("Please select size and style.");
return;
}
subtotal = subtotal * quantityval;
total += subtotal;
}
private void CalculateExtras()
{
if (echinaceacb.Checked)
{
extras += 0.75m;
}
if (beepollencb.Checked)
{
extras += 0.75m;
}
if (energyboostercb.Checked)
{
extras += 0.75m;
}
extras = extras * quantityval;
total += extras;
}
private void CalculateDiscount()
{
if (couponrb.Checked)
{
discount = total * 0.1m;
}
else if (preferredrb.Checked)
{
discount = total * 0.15m;
}
total -= discount;
}
private void AddTax()
{
tax = total * 0.08m;
total += tax;
}
private void displayAll()
{
subtotallbl.Text = subtotal.ToString("C");
extraslbl.Text = extras.ToString("C");
discountlbl.Text = discount.ToString("C");
salestaxlbl.Text = tax.ToString("C");
amountduelbl.Text = total.ToString("C");
}
private void exitbtn_Click(object sender, EventArgs e)
{
this.Close();
}
private void clearbtn_Click(object sender, EventArgs e)
{
}
private void aboutbtn_Click(object sender, EventArgs e)
{
}
private void exitToolStripMenuItem_Click(object sender, EventArgs e)
{
this.Close();
}
}
}
>>54292
?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54296
>>54295
you type bad numbers and see if you get an error
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54297
>>54296
oh, yeah
tested and passed
just need the math now
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54298
>>54293
those are reassigned each time you click the button
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54299
>>54298
are you sure? he said they were stacking
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54300
>>54297
I think you didn't check for the quantity to be greater than 0.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54301
>>54293
>>54298
Some of these get recalculated so in theory it shouldn't matter? But! discount definitely needs to get set to zero at some point, otherwise the user may use a discount once, and then tit will remain set for later purchases.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54302
>>54298
not all of them are, I mean, and he can just move them all to the end since that seems cleaner, right?
>>54300
he removed that when I added it, he's a big bully
>>54301
>>54287
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54303
>>54302
Hehe, I missed that one xwx
You were correct and Monsterboy should have listened! Bad boy!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54304
>>54300
this is the sample he gave me, he didn't even account for that, so I am not too worried
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54305
>>54299
oh, that. I would have keet them local to their individual funs
private void CalculateExtras() {
decimal extras = 0m;
...
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54306
>>54297
Also don't forget to implement the Clear button ^.^''
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54307
>>54306
it's okay, he can do that after because the actual values used to calculate shouldn't be cleared with the clear button, but before that, just in case someone calculates more than one time before clearing
I'm sure he won't forget~
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54308
>>54307
>just in case someone calculates more than one time before clearing
Well, if they get recalculated with clicking the button anyways, then it doesn't matter, yeah.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54309
you know I think this sample was really well thought out
the extras are only counted once I think
>>54306
oh, yea
I think I got that
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54310
>>54309
can we see your code once more please~?
would you like it to calculate the extras one time, or many?
it seems like an oversight on their part, but it's up to you, really
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54311
>>54309
>the extras are only counted once I think
Well, in the sample they are, but that feels wrong, no? You order them for each smoothie, not just one yeah? And so you should pay for all of it.
I think you could keep it the way you have it now, and add a comment inside the relevant part of the code that you did that on purpose and that if it was meant to do what the sample picture shows, you would just remove the part where you multiply the extras by the quantity, yes?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54312
I'm happy for you monster-anon, good job getting it all working~
I'm sorry that I'm not so good but at least I was able to help, I had fun!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54313
remember to read McConnell's book, it's popular and easy to find
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54314
sorry, my mother had to order pizza
>>54310
ummm, here you are again if you want
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Smoothie_Queen_Calculator___Final_Project
{
public partial class Form1 : Form
{
int quantityval;
decimal subtotal;
decimal extras = 0M;
decimal discount = 0M;
decimal tax;
decimal total;
decimal[,] smoothieListDecimal = {{ 5.99M, 6.99M, 7.99M, 8.99M },
{ 6.99M, 7.99M, 8.99M, 9.99M }};
public Form1()
{
InitializeComponent();
}
private void calculatebtn_Click(object sender, EventArgs e)
{
string customername = customernametxt.Text;
if (customername.Length == 0)
{
MessageBox.Show("Please enter the customer name");
return;
}
bool tempval = Int32.TryParse(quantitytxt.Text, out quantityval);
if (!tempval)
{
MessageBox.Show("Please enter a numeric quantity");
return;
}
total = 0m;
CalculateSubTotal();
CalculateExtras();
CalculateDiscount();
AddTax();
displayAll();
}
private void CalculateSubTotal()
{
if (sizecb.SelectedIndex != -1 && stylecb.SelectedIndex != -1)
{
subtotal = smoothieListDecimal[stylecb.SelectedIndex, sizecb.SelectedIndex];
}
else
{
MessageBox.Show("Please select size and style.");
return;
}
subtotal = subtotal * quantityval;
total += subtotal;
}
private void CalculateExtras()
{
if (echinaceacb.Checked)
{
extras += 0.75m;
}
if (beepollencb.Checked)
{
extras += 0.75m;
}
if (energyboostercb.Checked)
{
extras += 0.75m;
}
extras = extras * quantityval;
total += extras;
}
private void CalculateDiscount()
{
if (couponrb.Checked)
{
discount = total * 0.1m;
}
else if (preferredrb.Checked)
{
discount = total * 0.15m;
}
total -= discount;
}
private void AddTax()
{
tax = total * 0.08m;
total += tax;
}
private void displayAll()
{
subtotallbl.Text = subtotal.ToString("C");
extraslbl.Text = extras.ToString("C");
discountlbl.Text = discount.ToString("C");
salestaxlbl.Text = tax.ToString("C");
amountduelbl.Text = total.ToString("C");
}
private void exitbtn_Click(object sender, EventArgs e)
{
this.Close();
}
private void clearbtn_Click(object sender, EventArgs e)
{
}
private void aboutbtn_Click(object sender, EventArgs e)
{
}
private void exitToolStripMenuItem_Click(object sender, EventArgs e)
{
this.Close();
}
}
}
idk
>>54311
>but that feels wrong,
yea, I don't know what I want to do about that
>>54312
~
thank you very much poppy, and you too mouse, and the 'holo" anon as well
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54315
>>54313
thank you for helping me help him, neat holo anon~
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54316
>>54314
no problem~ it wouldn't be good if you had to pay someone, and I think you would have done that instead of learning, so that wouldn't be very good, I think
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54317
>>54316
nah, I wouldn't learned anything tbh
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54318
>>54313
>>54314
I'm sure it must be a mistake on their part, it wouldn't make sens to charge for the extras only once.
private void clearbtn_Click(object sender, EventArgs e)
{
subtotallbl.Text = "0";
extraslbl.Text = "0";
discountlbl.Text = "0";
salestaxlbl.Text = "0";
amountduelbl.Text = "0";
//tick off the extras
//set no discount
//any other stuff?
}
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54319
>>54318
>//any other stuff?
Oh, of course, clear the namefield, clear the quantity, clear / set to default the size and style! And bring focus to the customer name, as per >>53827
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54320
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Smoothie_Queen_Calculator___Final_Project
{
public partial class Form1 : Form
{
int quantity;
decimal total;
decimal[,] smoothieListDecimal = {{ 5.99M, 6.99M, 7.99M, 8.99M },
{ 6.99M, 7.99M, 8.99M, 9.99M }};
public Form1()
{
InitializeComponent();
}
private void calculatebtn_Click(object sender, EventArgs e)
{
string customername = customernametxt.Text;
if (customername.Length == 0)
{
MessageBox.Show("Please enter the customer name");
return;
}
if (Int32.TryParse(quantitytxt.Text, out quantity))
{
MessageBox.Show("Please enter a numeric quantity");
}
else
{
quantity = 0;
}
CalculateSubTotal();
CalculateExtras();
total = total * quantity;
CalculateDiscount();
AddTax();
displayAll();
}
private void CalculateSubTotal()
{
if (sizecb.SelectedIndex != -1 && stylecb.SelectedIndex != -1)
{
total = smoothieListDecimal[stylecb.SelectedIndex, sizecb.SelectedIndex];
}
else
{
MessageBox.Show("Please select size and style.");
total = 0m;
}
}
private void CalculateExtras()
{
decimal extras = 0m;
if (echinaceacb.Checked)
{
extras += 0.75m;
}
if (beepollencb.Checked)
{
extras += 0.75m;
}
if (energyboostercb.Checked)
{
extras += 0.75m;
}
total += extras;
}
private void CalculateDiscount()
{
decimal discount = 0m;
if (couponrb.Checked)
{
discount = total * 0.1m;
}
else if (preferredrb.Checked)
{
discount = total * 0.15m;
}
total -= discount;
}
private void AddTax()
{
total += total * 0.08m;
}
private void displayAll()
{
subtotallbl.Text = subtotal.ToString("C");
extraslbl.Text = extras.ToString("C");
discountlbl.Text = discount.ToString("C");
salestaxlbl.Text = tax.ToString("C");
amountduelbl.Text = total.ToString("C");
}
private void exitbtn_Click(object sender, EventArgs e)
{
this.Close();
}
private void clearbtn_Click(object sender, EventArgs e)
{
}
private void aboutbtn_Click(object sender, EventArgs e)
{
}
private void exitToolStripMenuItem_Click(object sender, EventArgs e)
{
this.Close();
}
}
}
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54321
>>54320
wat is this
>>54318
I think you mean
private void clearbtn_Click(object sender, EventArgs e)
{
subtotallbl.Text = "$0.00";
extraslbl.Text = "$0.00";
discountlbl.Text = "$0.00";
salestaxlbl.Text = "$0.00";
amountduelbl.Text = "$0.00";
//tick off the extras
//set no discount
//any other stuff?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54322
>>54320
oh fug
if (! Int32.TryParse(quantitytxt.Text, out quantity))
{
MessageBox.Show("Please enter a numeric quantity");
quantity = 0;
}
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54323
>>54321
oh nevermind, I am just going to go with poppy's, the way they did just seems wrong
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54324
>>54320
if (Int32.TryParse(quantitytxt.Text, out quantity))
{
MessageBox.Show("Please enter a numeric quantity");
}
else
{
quantity = 0;
}
Why is there the else quantity = 0; ?
>>54322
Ah, was a mistake eh?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54325
>>54321
clarification, optimization; let me put it with the correction
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Smoothie_Queen_Calculator___Final_Project
{
public partial class Form1 : Form
{
int quantity;
decimal total;
decimal[,] smoothieListDecimal = {{ 5.99M, 6.99M, 7.99M, 8.99M },
{ 6.99M, 7.99M, 8.99M, 9.99M }};
public Form1()
{
InitializeComponent();
}
private void calculatebtn_Click(object sender, EventArgs e)
{
string customername = customernametxt.Text;
if (customername.Length == 0)
{
MessageBox.Show("Please enter the customer name");
return;
}
if (! Int32.TryParse(quantitytxt.Text, out quantity))
{
MessageBox.Show("Please enter a numeric quantity");
quantity = 0;
}
CalculateSubTotal();
CalculateExtras();
total = total * quantity;
CalculateDiscount();
AddTax();
displayAll();
}
private void CalculateSubTotal()
{
if (sizecb.SelectedIndex != -1 && stylecb.SelectedIndex != -1)
{
total = smoothieListDecimal[stylecb.SelectedIndex, sizecb.SelectedIndex];
}
else
{
MessageBox.Show("Please select size and style.");
total = 0m;
}
}
private void CalculateExtras()
{
decimal extras = 0m;
if (echinaceacb.Checked)
{
extras += 0.75m;
}
if (beepollencb.Checked)
{
extras += 0.75m;
}
if (energyboostercb.Checked)
{
extras += 0.75m;
}
total += extras;
}
private void CalculateDiscount()
{
decimal discount = 0m;
if (couponrb.Checked)
{
discount = total * 0.1m;
}
else if (preferredrb.Checked)
{
discount = total * 0.15m;
}
total -= discount;
}
private void AddTax()
{
total += total * 0.08m;
}
private void displayAll()
{
subtotallbl.Text = subtotal.ToString("C");
extraslbl.Text = extras.ToString("C");
discountlbl.Text = discount.ToString("C");
salestaxlbl.Text = tax.ToString("C");
amountduelbl.Text = total.ToString("C");
}
private void exitbtn_Click(object sender, EventArgs e)
{
this.Close();
}
private void clearbtn_Click(object sender, EventArgs e)
{
}
private void aboutbtn_Click(object sender, EventArgs e)
{
}
private void exitToolStripMenuItem_Click(object sender, EventArgs e)
{
this.Close();
}
}
}
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54326
>>54321
Aha, yeah probably!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54327
>>54324
>Why is there the else quantity = 0; ?
Oh, I see now this wasn't the only thing wrong, it was reverse wasn't it.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54328
in clearbutton click, you can set the values to zero, and then run UpdateValues();
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54329
and then blank the name field and the radio buttons and such
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54330
>>54321
>>54325
the Extras stack for some reason
>>54329
I was just planning setting everything back to the way it was
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54331
>>54330
They are supposed to stack, no? If you have one ticked, 0.75$, if two, 1.50$ etc.
Oh wait, you don't set them to zero in the procedure (like Holoposter does).
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54332
>>54325
the way you're defining the tax and the discount and stuff inside of the functions, how will displayAll see it?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54333
>>54331
like I did from the start, this is why so many different code edits is confusing
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54334
>>54331
one click of the cal button is just going to be one order
two clicks have nothing to do with each other
pls tell me how to fix
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54335
>>54330
I forgot, this is c#. the original was better although I think setting the total to 0 in case of error is better than ignoring it.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54336
>>54334
I told you how to fix
private void UpdateValues()
{
subtotallbl.Text = subtotal.ToString("C");
extraslbl.Text = extras.ToString("C");
discountlbl.Text = discount.ToString("C");
salestaxlbl.Text = tax.ToString("C");
amountduelbl.Text = total.ToString("C");
subtotal = 0m;
extras = 0m;
discount = 0m;
tax = 0m;
total = 0m;
}
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54337
>>54332
that was the point, I forgot you want displayed
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54338
okay, now it isn't for some reason
this shit man
>>54336
yeah, I went back up, thank you lots~
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54339
>>54337
o-oh, oki, sorry, that's the first mistake I've seen you make, so I thought it was me being silly~
>>54338
no problem! let me know if there's any other issues please
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54340
>>54333
Fair enough!
>>54334
>one click of the cal button is just going to be one order
>two clicks have nothing to do with each other
I realize.
For the record, not to bully Poppy, but I think it would be better to set extras to 0 with every call of CalculateExtras(), at the beginning of the procedure x:
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54341
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54342
>>54340
like I said, all the edits make it confusing, I had the stuff being set to zero at the start of each part originally, but I changed it, you can set them to zero when defining them and then set them to 0 again at the end of the operation
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54343
>>54340
I am just going to upload the one that doesn't account for multiple smoothie extras, then e-mail a one that does, and ask him about it
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54344
the only thing that wasn't set to zero at the start was tax, because tax is set, not added to
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54345
>>54340
Now that you meantion it, extras is never initialized explicitly. Good thing c# does it to 0, but this wouldn't work in other languages.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54346
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54347
>>54346
hello~ how are you today? happy that it's the weekend now?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54348
>>54343
by the original, i mean your version, just with setting numbers to 0 in case of error
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54349
>>54343
I would do it the other way around… though! If they use an automated thing to check for inputs and outpu- no wait, they can't be doing that with the GUI can they?
Well, idk, but sending the email should cover your bases.
>>54345
>>54344
Woop, something to fix!
>>54346
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54350
>>54349
what do you mean? what's not fixed?
tax isn't added to, it's set.
the other thing isn't an issue.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54351
>>54347
I'm fine.
Not very enthusiastic about the weekend, no.
>>54349
What are you tagging me for?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54352
>>54349
wait, yes it is initialized
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54353
shit, does anyone know the right phrase to uncheck uncheck check boxes and radio button
>>54348
I am fine with it as is, don't want to fucking something up
>>54349
because it isn't in the sample he gave me, but I guess I can do that
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54354
>>54353
you didn't keep my changes I hope
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54355
>>54350
Woops, a mistake. Just the extras.
>>54350
>>54352
Oh never mind then!
>>54351
Guess I'm trying my luck.
>>54353
Either way should be fine.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54356
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54357
>>54354
nah, I have 6 back-ups at all times
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54358
>>54355
Luck at what, I wonder.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54359
>>54358
Luck at how far your common courtesy extends.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54360
>>54356
>nevermind
Does that mean you solved it?
Can you post the clearbutton now?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54361
>>54360
umm, no, I thought I had it, but doesn't seem like it want to work
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54362
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54363
>>54361
button.Checked = false; no?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54364
>>54362
Have you had a hard day?
>>54363
Suspiciously simple.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54365
>>54363
>>54361
Googling makes it seem like Holo is right though. At least for the checkboxes it should work?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54366
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54367
>>54364
I was just paid 600USD to go to school, wear a uniform, and do pushups; I have nothing to complain about.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54368
>>54365
oh, was putting in the wrong name
here is the button
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54369
>>54368
almost forgot
customernametxt.Text = "";
-_-
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54370
>>54366
That settles that then.
>>54367
Not excited about the weekend though, mmm?
>>54368
Do the comboboxes / dropdowns work properly if you clear them with the button?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54371
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54372
>>54370
>>54370
yeah, seems too
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54373
>>54370
>>54372
it's just syntactic sugar, you're actually calling a function and that takes care of everything
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54374
>>54371
I just thought you would, after a long week. Sorry.
>>54372
Good.
>>54373
Ooooh, I see. What's the wording. The = operator is overloaded for that variable? Is that a thing?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54375
>>54374
Syntactic sugar. = is not an operator.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54376
>>54374
hey, do you know how to open a form with a button. I thought I did, but now I don't
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54377
>>54375
I was thinking of something else. It is an operator. It's even overloadable in c++ but who would do that.
>>54376
uh
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54378
>>54375
Is it not?
>>54376
I don't know either. What about that Click.calculatebtn, that's reverse, isn't it?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54379
>>54378
that is just the drop down menuStrip
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54380
>>54374
There's nothing to really look forward to about the weekend, so
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54381
>>54377
>but who would do that
I thought it was nifty?
>>54379
I am reasonably certain the dropdown menu should be possible to make in the GUI. But you wanted the form to appear when you click on About, yeah?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54382
>>54380
A mark of someone who has no one to try their luck on.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54383
>>54381
Idk, I was just starting on it, when I found I couldn't get the about button to work
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54384
>>54382
Aren't you still single?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54385
>>54381
it makes the syntax even more complicated
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54386
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54387
>>54383
Ahh, I see.
So you designed the about form in the gui and now want it to pop up on click?
>>54384
I am. Want to try your luck?
>>54385
But it allows you to
1) make things more convenient for you, and
2) when making a library for someone else, you can take measures and make it more dumbproof by doing whatever checks you need to make sure he does not assign stuff wrong. You can throw errors / exceptions and suchlike, yeah?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54388
>>54387
Never would have guessed.
No thanks.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54389
>>54387
yeah, I just added another form, and want it to pop up when the aboutbtn is clicked
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54390
>>54387
= is for shallow copies. That is all it should do.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54391
>>54388
I wasn't making you?
>>54389
Hmm. Idk why what you wrote doesn't work. I guess you could try putting
AboutForm About;
among the variables
and then into the constructor
public Form1()
{
InitializeComponent();
About = new AboutForm();
}
But I don't see why that would help.
>>54390
Umm, o-okay.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54392
>>54391
found out it, do you know how to make menuStrip click things?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54393
>>54391
w-what? did it seem like he thought you were making him?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54394
>>54392
You mean the dropdown menu? Like when in applications you have, say, File, View, Properties, and so on? Again, I think that part should be doable via the gui.
>>54393
I don't see where the question came from, that's all.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54395
>>54394
nah, I just need these two to click the same buttons
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54396
>>54395
Couldn't you just put something like
calculatebtn_Click(sender, e)
or maybe
this.calculatebtn_Click(sender, e)
into the <menuitem>_Click
Anyway. I am going to bed. Wish me good night, /tripfriends/.
Good night, /tripfriends/
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54397
>>54396
good night mouse
sleep well
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54398
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54399
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54400
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54401
>>54400
sleep well monster anon
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54402
Rude awakenings because someone is torching shit suck.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54403
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54404
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54405
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54406
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54407
>>54405
confluent if it finishes, but not decidable
>>54406
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54408
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54409
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54410
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54411
>>54409
hello poppy, it is good to have you back
>>54410
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54412
>>54411
h-hello, really? thank you
did you have some of your cereal this morning?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54413
>>54412
I mean from moving.
I had carrots
how is your day going?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54414
>>54413
I had really bad dreams, but I think it's going okay
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54415
>>54414
I have been watching youtube and drawing, as I don't have to worry about school anymore
have darkest dungeon, so that is going to be fun
do you have anything you want to get done today?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54416
>>54415
I'm not sure, I've been feeling really weird recently
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54417
>>54416
are the bad dreams part of it?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54418
>>54417
no, m-maybe a cause of the bad dreams, but not the other way around, I think
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54419
>>54418
do you have an idea what it is?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54420
>>54419
probably just moving, I really don't like moving
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54421
>>54420
must suck having to deal with every year then, and to take so long in doing it.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54422
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54423
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54424
>>54405
I don't remember what those are and am too tipsy to be reading it.
I am pretty much positive I don't know what confluent and decidable means (in the context of programming).
>>54422
Lewd.
>>54421
Hello. So are you finished with the program?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54425
>>54424
yea, submitted, and done
:D
hello mouse, how is your day going?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54426
>>54425
Good to hear ^.^
Did you figure out the About form (I think you did), and making the menu options do what clicking the button does?
Oh it's been alright. Had family visits the whole afternoon, now they are gone. Am pretty tipsy.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54427
>>54426
yep, all done, just like the simple
I might be visiting family as well today
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54428
File: 9ddbc8beab181e6⋯.jpg (Spoiler Image,187.73 KB,1003x1417,1003:1417,9ddbc8beab181e67f46ae8cecb….jpg)

>>54427
Good job! And without cheating ^.^
Good luck with it.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54429
>>54428
well, poppy did most of the work
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54430
>>54429
That is fair to admit, yeah.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54431
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54432
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54433
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54434
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54435
>>54431
Well it is done so there!
>>54433
Hello hello.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54436
>>54435
I know, I have stressed about it for awhile
did you enjoy your family visit?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54437
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54438
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54439
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54440
>>54436
Yeah.
Oh yeah, wasn't bad. I only hid in my room for maybe an hour or 90 minutes, which isn't too bad when you consider people have been here from like 12 till 7pm.
You had fun relaxing?
>>54437
>>54439
So what's up Holo?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54441
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54442
>>54440
>I only hid in my room for maybe an hour or 90 minutes
you don't live alone, do you?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54443
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54444
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54445
>>54444
So what's up, quadsholo?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54446
>>54443
okay, otherwise, that would have very odd
>>54444
nice 4s
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54447
>>54445
I misremembered this
yosefk.com/c++fqa/ctors.html#fqa-10.19
and thought it showed the grammar is not confluent
>>54446
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54448
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54449
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54450
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54451
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54452
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54453
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54454
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54455
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54456
>>54446
Well yeah of course.
>>54447
I take it you are okay.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54457
I have to go do something
>>54455
>>54456
be back in a bit
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54458
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54459
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54460
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54461
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54462
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54463
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54464
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54465
>>54464 nice pic, hehe
>>54463
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54466
>>54465
didn't I say I wasn't to be lewd with your anymore?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54467
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54468
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54469
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54470
>>54469
So will I…. not to mention I will be playing a game anyways.
>>54468
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54471
>>54470
I also have a game to play
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54472
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54473
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54474
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54475
>>54474
nice thing~ what's that? a hat and something else?
>>54473
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54476
>>54475
yeah, I was in a laundromat, and playing around on my phone
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54477
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54478
>>54476
I see, no washing stuff in your new house? wawa
>>54477
hello, are you going to become a eurosleeper now?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54479
>>54478
It's 3am, I probably should
I don't know though.
How are you?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54480
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54481
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54482
>>54481
>>54480
Just booping you to say good night, really.
Good night Monsterboy ^.^
>>54478
And good night to you as well, Poppy-chan~
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54483
>>54482
Good night mouse
Rest well
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54484
>>54479
I'm okay, just spending some time doing stuff so time passes nicely
>>54482
good night, see you next time
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54485
I hope I die
I'm sorry maddex
Have a Christmas mado
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54486
I'm sorry I used you as an outlet for my affection for noun. I'm sorry I let it ruin our friendship I'm sorry I tormented you. Idk you probably don't give a shit but I hope the best for you. You're smarter than me you'll go farther than me. I'm sorry I was so horrible I really do think I loved you and I'm sorry I can't control myself. I'm sorry
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54487
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54488
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54489
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54490
"if you dont got no sauce then you lost, but you can also get lost in the sauce" - Chef Boiardi
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54491
>>54490
so how you doing, mad?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54492
>>54491
doing p damn great.
my chess set came in today and it surpassed my expectations
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54493
>>54492
why did you get that?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54494
>>54493
Cause I play Chess. duh
It was only $30 with 2 day delivery. It looks nice af and its nice to use.
I've never got in to Chess big time, but I plan to get involved in the community more.
Buying my own set gives me a social outlet, or so I hope.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54495
>>54494
well, i hope that works out
anyway, are you any good at it?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54496
>>54495
Im decent at best rn. But Im rusty as well as I never had much experience to begin with
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54497
>>54496
you can always test yourself against a computer
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54498
>>54497
>test yourself against a computer
those bots are designed to analyze every possible move you can make and counterplay it
they're not natural to play against and they are a personal taboo to me
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54499
>>54498
they are the best players, so if you can beat them, you'll be the best player
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54500
>>54499
no they're not
cause they arent a player to begin with
and they play the game systematically. give it time and you can beat the bot's ass 100-0
a person has personal flair to their playstyles. its not as easy to study something so arbitrary
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54501
>>54500
a computer beat the best chess player in the world
anyway
good night, I hope you find many friendships with your plan
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54502
I stole a credit card. I'm going to buy a bunch of shit do a bunch of drugs and jump off a bridge i love you maddex I'm sorry idk why sinced I robotripped I can't stop thinking of you I hate this idc I just wanted to talk to you I really hope the best for you
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54503
>>54502
>druggie jumping off a bridge
Could it be?
Is this the day my memes finally come true?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54504
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54505
>>54502
Don't forget to do a flip.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54506
>>54502
Stop bluffing and do it faggot, don't foget to record it tho.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54507
>>54505
I think I recall your character, as in your persona here
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54508
man Im two much for these niggas, and three much for these hoes
I got the world in my hands and I keep my hands closed
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54509
now get off my dick I aint fuckin wit ya
watch me shoot to the bank IM A MONEY PISTOL
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54510
>>54507
I'm not trying to hide it; I've merely found a new flavor of the week to post with.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54511
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54512
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54513
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54514
>>54513
your day going well?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54515
>>54514
Mhmm. Drinking early in the afternoon.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54516
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54517
>>54502
Send us the 4x4 you get in page 12 of your local newspaper when you wake up
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54518
>>54516
Pretty good, yeah. Although we opened it yesterday. I covered the bottle with a food wrap so I don't think it went stale too much, only thing is we kept it in the fridge so it is way too cold, for now.
How are you doing today?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54519
>>54518
just woke up, my hand feels odd.
because I got a new bed, I don't move around as much, so the blood in my hand doesn't have to change very much
making my hand feel numb and cold
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54520
>>54519
Oh. All the best to your hand.
Hmm. I need to walk the hound now. Be back in 20-30. See you soon Monsterbee.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54521
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54522
>>54521
Yuss. Almost perfectly in the middle. Hello hello.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54523
>>54522
did you have fun with your dogo
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54524
>>54523
Eh, it was a doggo alright. What are you up to?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54525
>>54524
drawing, going to play a game
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54526
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54527
good morning everyone, having a nice first weekend of december~?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54528
>>54527
morning poppy
>>54526
so, you have any plans for today, beside drinking
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54529
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54530
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54531
>>54529
did your school go well?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54532
>>54531
It still is. Reasonably so. Just reading a piece of literature now, that's not really anything I need to take notes on, so it's fairly fun.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54533
>>54532
well that is good
I remember they had you reading something that made no sense
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54534
>>54533
Hmm. Finished it now. Wonder what more there is to read.
I guess you mean "The origin of the family, private property, and the state"
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54535
>>54534
yeah, that just sounds useless and convoluted
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54536
>>54535
I took like 15 days to read it, even though it was only about 110 pages long. It is strange though, it wasn't uninteresting, really. It did contain a good amount of historical observations. It was a reasonably good introduction into how societies used to organise themselves before what we know today.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54537
>>54536
>It was a reasonably good introduction into how societies used to organise themselves
Well, I said that as if I knew a lot about the topic and was able to tell what is and what is not a good introduction to that topic. Which I do not. So…. take with a grain of salt, I suppose.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54538
>>54536
>>54537
well, you know more about it then I do
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54539
>>54538
Who knows. It's interesting to look at things that you take almost completely for granted and see how foreign they are or were in other cultures.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54540
>>54539
I have never really been a big fan of that kind of thing.
biology > economics > culture
culture is at the end of all of this, imo
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54541
>>54540
I think it might only be at the end for you because you are so deeply immersed into it.
I'm not saying the study of culture is science, mind you.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54542
>>54541
>because you are so deeply immersed into it
umm… I am pretty sure I am much as a layman as you are, mouse
>I'm not saying the study of culture is science, mind you.
so are your finals coming up? mine are.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54543
>>54542
are you confident?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54544
>>54542
>umm… I am pretty sure I am much as a layman
I meant culture itself, not the study of culture.
Hmm. I only know about the date of one class's finals, and that is 5th January.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54545
>>54543
I am going to cheat, yea
the only one I might not pass is Principles of Macroeconomics, and that isn't because I did poorly on in of the tests, it is because he does post the discussions to the course calendar, so I don't know what I missed
>>54544
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54546
>>54545
Mmmm?
Also, nice numbers.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54547
>>54546
I advocated euthanizing terminally ill people passed a certain age as way to cut cost, so they wouldn't be such a burden on a economy, so I don't think the professor likes me
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54548
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54549
>>54548
anyway, I'll be around, have a good day mousei~
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54550
>>54549
Thank you, you too ^.^
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54551
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54552
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54553
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54554
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54555
>>54554
Hey hey. How are you doing, sir?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54556
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54557
File: b794588cb1b393e⋯.jpg (Spoiler Image,338.52 KB,1058x2046,529:1023,b794588cb1b393ec4b0c38d08b….jpg)

>>54556
"I am crying, but wet, Mouse."
Okay!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54558
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54559
File: 97614b79d60e182⋯.jpg (Spoiler Image,455.83 KB,595x842,595:842,97614b79d60e1824d013a87cf7….jpg)

Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54560
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54561
>>54560
That RJ is oddly smutty even though there is no nudity.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54562
>>54561
she's from the Umaru anime
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54563
>>54562
Oh? Looks like Ryuujou to me :thinking:
I guess you now better. You are the bigger weeb between the two of us after all.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54564
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54565
>>54564
Still a bigger weeb than me.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54566
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54567
>>54563
you're always thinking silly things
>>54565
w-waw, bullied
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54568
>>54566
Ya-huh.
>>54567
Thinking or :thinking: ?
Me? How?!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54569
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54570
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54571
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54572
YouTube embed. Click thumbnail to play.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54573
mouse say's i broke it but he is just being mean
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54574
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54575
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54576
>>54575
searching adeleine on google image's is not a good idea
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54577
>>54576
Is that right?
You haven't been here for a while.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54578
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54579
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54580
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54581
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54582
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54583
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54584
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54585
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54586
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54587
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54588
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54589
>>54588
Rock, you are a rock.
What's the next verse?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54590
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54591
>>54590
I didn't expect there to actually be another verse.
Holo anon.
You are Holo anon.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54592
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54593
>>54592
Cheeky and a nerd,
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54594
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54595
>>54594
Just like the neat bird.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54596
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54597
>>54596
Shouldn't have called you anon, I mean, what the hell rhymes with it?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54598
>>54597
kurenai, impefectly
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54599
>>54598
I was looking for a more accurate rhyme
>crimson
though I must admit I didn't come up with anything better than that.
Holo, you are Holo.
Cheeky and a nerd,
just like the neat bird.
Hashtag YOLO.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54600
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54601
>>54600
It's in the ballpark of the original Maud poem.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54602
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54603
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54604
>>54602
You write one now.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54605
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54606
>>54604
The plane is coming down
You face disaster
There's no feather in your crown
Your prize is lost to the big guy master
>>54605
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54607
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54608
>>54605
Wew.
>>54606
Not bad, although I meant:
write one about you.
A stanza for a penny, or a cent,
what harm could that do?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54609
>>54607
looks like someone compiled people screaming at me
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54610
>>54609
Don't drive like an idiot then!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54611
>>54608
nah
>>54610
No, I'll make one from my point of view and counter the video by showing off my driving skills.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54612
>>54611
Eurobeat starts playing in the background
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54613
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54614
>>54611
Good night, anon.
And good night all ye tripfriends.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54615
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54616
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54617
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54618
>>54617
I'm gonna do a sleep now too, good night Anon
Good Night /tripfriend/
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54619
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54620
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54621
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54622
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54623
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54624
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54625
>>54623
Holo, the bold and the beautiful. How's it going, good ser?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54626
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54627
good morning again everyone~
having some of your cereal this morning monster-anon?
>>54623
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54628
>>54627
whoops, I forgot my name and flag, no one will ever be able to tell who I am without that!!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54629
>>54626
don't know if I should draw, or play the game
found a furry comic with minimal authropmorphization after I got done with today's homework
who is your day going
>>54627
hello, poppy
I ate a sandwich
doing well I trust?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54630
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54631
>>54628
Oh, it was Poppy-chan, who could have known?!
Hello hello.
>>54629
Toss a coin and if it lands and you don't feel like doing that thing, that means you wanna do the other one more.
Ah, is it fun?
Been fairly tiring. Oh well, I am home now.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54632
>>54629
yay, that's good. are you feeling any different through the day with breakfast in the morning?
y-you'll probably say no
>>54630
s-see?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54633
>>54631
good idea
Idk, I don't know how to feel about a zedra not kicking you to death if you tried to fuck it
>>54632
I ate two steaks, and lobster last night, so I don't know if it was that or the sandwich, but I havn't felt the need to eat anything today
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54634
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54635
YouTube embed. Click thumbnail to play.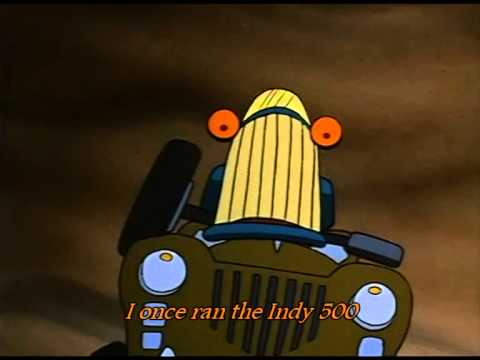
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54636
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54637
>>54632
I do now, phew!
>>54633
Ah, well, then just don't feel about it at all.
>>54636
Looks like 8chan is bullying you today.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54638
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54639
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54640
>>54637
I wasn't looking for it, it no reason to feel bad
I was looking for something else, and came across it, also found one in the style in lion king movie
work wasn't a bully for you today was it?
>>54638
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54641
>>54639
don't know how you are.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54642
>>54639
helloo~
how are you?
de geso
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54643
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54644
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54645
>>54638
I do see, that's what you asked, remember?
>>54639
Yin and yang, so presumably all is in balance! Not terrible, not great either, somewhere in a grey area.
>>54640
I'm not suggesting you were. If you were Holo anon, I might, but you're not so I am not.
Oh, a bit of a bully to be quite honest. The new guy didn't show up so I had to stand in for him, and whatnot.
Ah, be back in 20-30, got a dog to walk.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54646
>>54645
I-I think maybe you misunderstood what I was asking see about, silly mouse~
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54647
>>54645
>>54645
see you, then
It came up heads, so I'll be playing the game, so sorry If i don't back to you in timely fashion
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54648
>>54642
I'm fine desu. How are you?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54649
>>54646
Well, you either meant "See, someone didn't recognise me without my trip, exactly what I said!"
or "I activated my name and flag, I am Poppy-chan! See?"
Either of those work for me!
>>54647
I am seen again, hello.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54650
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54651
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54652
>>54651
what kind of doggo do you have anyway
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54653
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54654
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54655
>>54654
But old, poor feller. The second pic is from two or three years ago.
>>54648
>>54654
What up you guys?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54656
>>54655
looking for something to draw
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54657
>>54656
Hmmm. Well you just got two pics of a nice dog, hehe.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54658
>>54657
I guess you're right
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54659
>>54658
If you're comfortable doing it anyway.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54660
>>54659
of course I am
what are you up too, mouse
it must be 7 or 8 where you are
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54661
>>54660
Aha, oki.
Hmm, drank a smoothie and exercised, shitposting atm but I'm going to get something to eat and do some reading too.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54662
>>54661
I am watching ed edd n eddy while drawing your dog
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54663
>>54662
Aha, I wish you luck! And have fun. Poor fat catto.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54664
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54665
>>54664
how is your day going?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54666
>>54665
Lazily. It's almost about to end.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54667
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54668
>>54667
>>54667
Not sleeping yet baka. Kinda bored tho.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54669
>>54668
u said it was coming to an end tho
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54670
>>54669
>Almost
Implying it is the later part of my evening.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54671
>>54670
I was just saying for when the time comes
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54672
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54673
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54674
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54675
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54676
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54678
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54679
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54680
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54681
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54682
>>54681
>>54675
Could I also collect a good night? I will be going in like 10 minutes.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54683
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54684
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54685
>>54683
Night night Maddie.
>>54684
Night night Monsterbee ^.^ Hope you had fun with drawing.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54686
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54687
>>54686
look like you're only be able to talk to people over the weekend
good night, /tripfriend/
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54688
>>54687
Perhaps.
Sleep well.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54689
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54690
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54691
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54692
>>54691
I need to walk the hound before I fall asleep, rip.
See you in a few, Monsterbee.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54693
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54694
>>54693
How are you doing?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54695
>>54694
stole some dlc
how was your day
did the dude show up on time today?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54696
>>54695
Ehe. What dlc?
Kinda tiring.
Yeah he did. In fact he came an hour earlier by accident.
What you up to?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54697
>>54696
The Crimson Court and The Shieldbreaker
poor thing, you going to bed due to it?
still playing darkest dungeon
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54698
>>54697
Ahh, I see I see. Oh, darkest dungeon, I only heard of it, but when I looked it up, I realize I have seen the character illustrations before. Those are pretty nice.
Nah, not too soon anyway, thank you for the concern though ^^
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54699
>>54698
okay, then what are you planning to do today?
trying out another recipe?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54700
>>54699
Just more attempts to study, depending on how much disciplined I can muster. I don't like my chances…
What recipe?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54701
>>54700
the one with the pineapple you showed me a youtube video about
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54702
YouTube embed. Click thumbnail to play.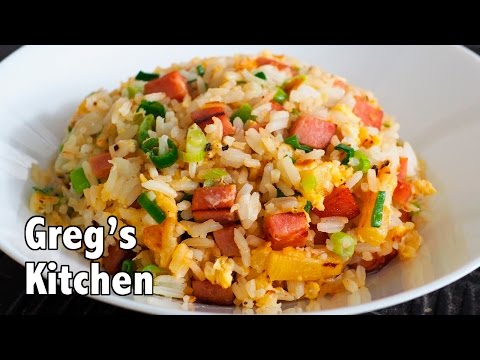
>>54701
Waaaait, this one? I showed you this?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54703
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54704
>>54703
it didn't turn out as good as you thought it would
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54705
>>54703
Aha, rats, I didn't even remember I did. Silly old me.
>>54704
Yeah, well that part I remember X3
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54706
YouTube embed. Click thumbnail to play.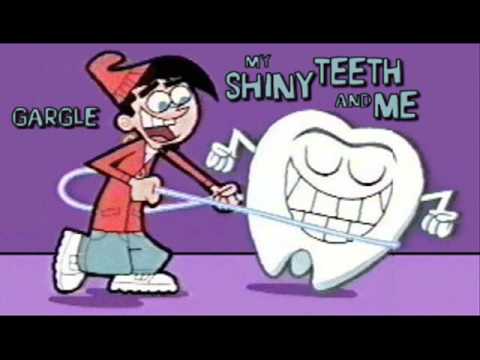
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54707
>>54706
Heheh.
I wonder if Holo anon is lurking.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54708
>>54707
he replies to your posts just to say hello, I am going with no
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54709
>>54708
Perhaps.
Also I forgot / missed your question.
>>54699
>okay, then what are you planning to do today?
In about 4.5 hours, I think.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54710
>>54709
well, I be around
have a nice day, mousei
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54711
>>54697
>still playing darkest dungeon
And damn, that game does look cool!
>>54710
Okay. You have fun, Monsterbabe.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54712
>>54711
>And damn, that game does look cool!
unforgiving as fuck tho, but you can always edit the files to make a little easier :3
you too
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54713
>>54712
Cheating! X3
Thank yous.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54714
your dog is hard to draw
too much fuff
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54715
>>54714
Aha, I suppose. He does have curly hair, which I imagine is harder than smooth-haired dogs are.
I need to go to bed now.
Good night, Monsterbee. Sweet dreams.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54716
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54717
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54718
>>54717
goodnight once more
poor old dog
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54719
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54720
>>54719
does he think you're old dog?
h-hello
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54721
>>54720
That appears to be the case, yes.
Good morning.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54722
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54723
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54724
>>54720
so he isn't the old dog
then who is he?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54725
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54726
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54727
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54728
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54729
>>54728
okay, adding it to the list
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54730
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54731
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54732
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54733
>>54732
oh you know
wake up, wish I hadn't, wish I died in my sleep, take little brother to school
try to finish drawing of your dog, didn't turn out good
watch youtube (invader zim), post on 8chan, came here, waited for you.
the life, I guess.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54734
>>54732
how is your day going?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54735
>>54733
Oh dear. The usual, I see.
Aha, if you don't want to show, I won't mind, but if you do, I won't laugh! Or if I do, I won't say it so you won't know! I'm not a bully though so I most likely won't.
>>54734
Aha, stupid day at work, but that's behind me now. Overtime and no time to have lunch, I hate these days (t-that's what kept me from replying earlier…eating and making coffee). I will be playing some game later tonight, in three hours or so, so that will be good.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54736
>>54735
I don't mind showing it, through the other one away already
well, at least you have something to ease the bullying of work
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54737
>>54736
I threw away the other one*
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54738
>>54736
Aha, that's not bad though It's not great but there is resemblance.
Aye. That and being here. No studying today, looks like, but when I come back tired and struggle to stay awake, reading yields little results anyway.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54739
>>54738
>>54738
>struggle to stay awake
have you been staying awake to long, or have the days been too hard
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54740
>>54739
A combination of both to be honest. I'm a dingbat and stay up too long.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54741
>>54740
>I'm a dingbat and stay up too long
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54742
>>54740
well, I hope you gain of good sleep tonight
I am going back to my game, so I'll be around.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54743
>>54741
Not sure what's supposed to be so black about the word dingbat.
>>54742
Thank you, hopefully.
Okiii, you have fun.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54744
>>54743
nah, the face. I keep this pic cause its someone that tried to shit talk me and its funny af
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54745
>>54744
>and its funny af
Is that right? >w>
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54746
>>54745
ye I mean like how are you entitled to talk shit if your face was smeared in it
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54747
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54748
>>54746
Naw man.
>>54747
Aha, cute ^.^
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54749
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54750
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54751
good morning~ it's still morning for me, so I'm in time~!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54752
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54753
>>54751
Hellu. Nice gif ^^
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54754
>>54752
hello monster anon~!
>>54753
hi, thank you~ it's an old poppy-use gif
>>54752
>>54736
y-you did a good job, but the way you do eyes scares me a little
m-maybe you should practise on making them less intense, if that's not your intention?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54755
>>54754
Aha, I see I see! Must be really old, I don't remember seeing it!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54756
>>54754
umm no
the dog was looking the person talking the picture
don't know why the eyes would look fearful
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54757
>>54756
they don't seem fearful to me, they seem very intense
I think you need to reduce the amount of shading under or around the eyes
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54758
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54759
>>54757
hmm, alright
I found a gesture youtube thing
:D
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54760
>>54759
>>54758
Good night Monsterboy, and good night Poppy-chan ^.^
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54761
>>54760
good night, mouse
sweet dreams
I hope you wake up well rested this time
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54762
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54763
no one is ever here anymore
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54764
>>54763
I-I think it's still the same, really
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54765
>>54763
you're not here over the weekends then
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54766
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54767
>>54765
Hello Monster.
>>54766
Beating a dead loli?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54768
>>54767
hello mouse
how is your day going?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54769
>>54768
Ahh,pretty okay. Still am a bit tired. I ordered a pizza though, and will make me a coffee now.
How has your day been?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54770
>>54769
my brother started riding this bus again, even tho we live 5 mins away from school, and they will be picking him up a hour early, and dropping him off an hour later.
and that is good for me, because I get 2 hours of not dealing with him, but stupid for the school
other then that, drawing or working up the gumption anyway
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54771
>>54770
Oh, I see.
Fingers crossed!
Woo, pizza arrived!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54772
>>54771
what kind of pizza?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54773
>>54772
mozzarella, spinach, chicken, garlic and cheese
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54774
>>54773
well, I hope you enjoy it
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54775
>>54774
Thank you. I was fairly hungry too, so it's good it came so soon.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54776
>>54775
is the pizza places you have there good
most here are mediocre at best
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54777
>>54776
I don't really know, I don't have means of comparing with other countries, ehe. I am guessing the same as yours.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54778
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54779
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54780
>>54777
so, do you have plans over the weekend?
>>54778
good morning?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54781
w-whoops, I forgot my image
>>54780
y-yes
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54782
>>54781
give mouse a (you) as well
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54783
>>54782
I had one at the end just blankly going to him but I erased it!!
>>54779
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54784
>>54783
what you doing today?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54785
>>54784
that's the best question ever
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54786
>>54785
are you saying that sarcastically or as in you do not know what you're doing today?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54787
>>54780
Well, my aunt's bf is celebrating his 70s and we have been invited so looks like that's gonna happen.
You?
>>54783
Whhyyyyyyyyy ;~;
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54788
>>54786
y-yeah, I'm not sure
I'm bored of studying for today, so I will just wonder what to do for a while, because I'm a silly.
what will you do today?
>>54787
why did I erase? b-because I thought you'd ask me why I sent a blank!!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54789
>>54787
>>54786
t-the conversational volleyball is on your side!!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54790
>>54787
I might go see family, I might finish up school for this semester.
My aunt got into meth via my cousin (her daughter) and she has been a bad influence on her for a long time now, however cousin might be going to jail due to a DUI and lying to the cops, and she has a history of drugs that the law knows about due to her being irresponsible in getting her children back.
the other cousins aren't bad tho, and my aunt has been taking advantage of one (who isn't 18 yet) for money, and rent.
mother has been going down there, and buying them groceries every other weekend, because aunt stopped doing it, so they are now relegated to walking to a fast food place. Might go down there and see what is going on. The 2 good cousins wanted to move out, and set up with their step dad last time I was down, but they don't know how to adult very well, so it is a slow process.
>>54788
and a cute
trying to force myself to draw, i have this odd block when I am trying to do things. it happens with everything, not just drawing
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54791
>>54789
it takes me a sec to write out things
I kind of want to order pizza now
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54792
>>54790
y-yeah, sometimes practising can be a little difficult, but at least it's on your mind~
>>54791
that's okay, I wasn't trying to rush you, sorry
t-that's not a good breakfast!!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54793
>>54792
I always have to be beholden to an entity that gives to due dates for me to be motivated, and the entity can't be myself for some reason ;_;
I'll always try to hit the conversational volleyball as fast as I can, or try to end in the game in a polite way after I run out of things to talk about
but it tastes good, and I don't have a car to do get anything with
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54794
>>54793
nice circle~ what happened to your cereal and sandwich stuff?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54795
>>54794
it is all gone, and I don't have corned beef, so sandwiches are out of the question
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54796
>>54793
and that's good of you to want to end stuff properly~
don't worry, I think I'm like that too, pleasing other people or meeting their goals or deadlines is easier than meeting my own
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54797
>>54795
you don't need beef for sandwiches, silly
just put some vegetables on toasted bread with some condiment that you like
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54798
>>54796
~
>>54797
I don't cheese I like, and no vegetables here, I don't think we have any lunch meat for that matter
I guess I could eat grapes and maybe a peanut butter and jelly sandwich
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54799
>>54798
y-yes, peanut butter and jelly is good
i-it sounds like you don't really ever have anything, what's happening?
d-d-d-does no one there buy any vegetables or cereal or anything?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54800
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54801
>>54798
that's a neat thing, what is it becoming?
>>54800
hello
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54802
>>54788
Maybe I would, but why does it matter?!
>>54790
Oh dear. Quite the messy situation.
How old are the cousins then?
>>54800
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54803
>>54799
my mother just buys things for my brother to get, and big that will feed people for days, but I am very picky and lazy
>>54801
a head
>>54802
one is 16 the other is going to be 18 soon, i think
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54804
>>54803
Ahh. For some that might be enough, but I am sure there are lots of people who aren't very adult at 18. Or 30.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54805
>>54804
what is even worse, it she fucked the almost 18 one out of free school, due to her lying on her taxes
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54806
>>54805
Ffffug, that is harsh :-/
I am falling asleep…. if I don't reply for a long time, probably failed to endure x__x
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54807
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54808
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54809
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54810
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54811
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54812
>>54808
>
>>54807
I am not going to sleep!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54813
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54814
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54815
>>54813
No no.
>>54814
Is the second button being unbuttoned canon? I wonder…
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54816
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54817
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54818
>>54816
Do you know?
>>54817
Nay nay.
Nice animu titties.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54819
>>54815
Hotaru's eyes are all wrong.
>>54817
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54820
>>54819
Was that the answer to the original question, or just whether you know the answer?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54821
>>54820
I don't know, I just know her eyes.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54822
>>54821
Her eyes are down there.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54823
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54824
>>54818
sorry, I was ummm. jerking off
yes
I don't want you losing sleep, mouse
>>54819
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54825
>>54823
Well those are all wrong too, aren't they?
>>54824
So you were, mhm!
No!
I am better now. It was partly because of the heat. Just opened the window and I'm better.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54826
>>54825
they are blue and the circles are represented by changing shades instead of lines
>>54824
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54827
>>54825
>heat
jeez, your heating system must be on point
>>54826
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54828
>>54826
The resulting impression is much more normal, not like the ones with the lines. Those look vaguely crazy or at least enthusiastic.
>>54827
Well it was cold here when I got back from work so I turned it to maximum and overdid it.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54829
>>54828
they're pretty
>>54827
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54830
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54831
>>54829
Pretty or not, she also looks crazy.
>>54830
Mhm!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54832
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54833
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54834
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54835
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54836
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54837
nicely toasted bread is just the best
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54838
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54839
>>54837
and corned beef
>>54838
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54840
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54841
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54842
>>54836
>>54837
Enjoy! ^.^ Do you have it with something?
>>54838
Well, that one looks just adorable (and maybe a bit confused).
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54843
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54844
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54845
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54846
YouTube embed. Click thumbnail to play.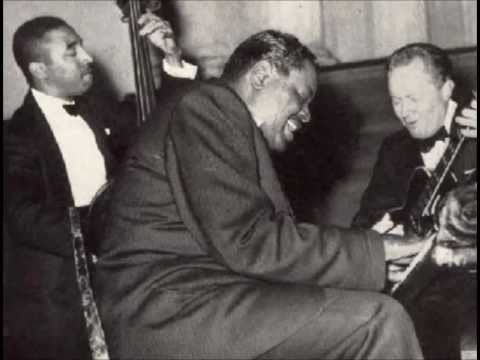
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54847
>>54843
^^
>>54844
Of course it is! L-like I didn't know that, pah.
>>54846
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54848
>>54842
j-just bread! I was trying some new bread
>>54843
sorry monster-anon!
>>54844
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54849
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54850
>>54846
hello
>>54847
~
>>54848
I forgive you
corned beef is still the best tho
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54851
>>54848
Ahhh, I see I see. Do you eat butter at all? I don't know about these things.
>>54849
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54852
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54853
>>54851
is that how you get a loli gf?
>>54852
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54854
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54855
>>54854
was work a bully on you too
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54856
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54857
YouTube embed. Click thumbnail to play.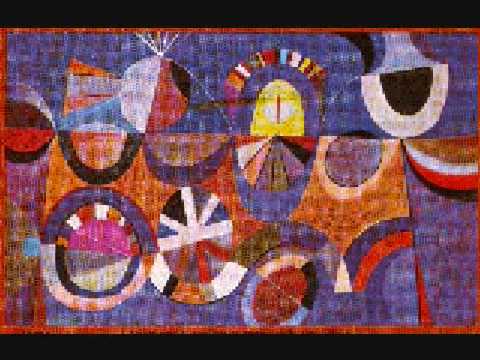
>>54855
Yhea, had to do some extra work again to relief someone so I was working for 5.5 hours in the cold rain and wind.
>>54856
Margarine?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54858
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54859
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54860
>>54850
Nice gif X3
>>54853
Probably.
>>54854
Going to bed early tonight?
>>54856
Ahh, that would have been my guess if I had to guess, but I didn't so it wasn't!
>>54858
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54861
YouTube embed. Click thumbnail to play.
>>54858
Hello, Holo. I'm eating pepernoten/kruidnoten (peppernuts/spicenuts) :3
>>54859
No fats at all to make the bread less try and help condiments is that the right word? stick?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54862
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54863
>>54861
b-bread is dry to you?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54864
YouTube embed. Click thumbnail to play.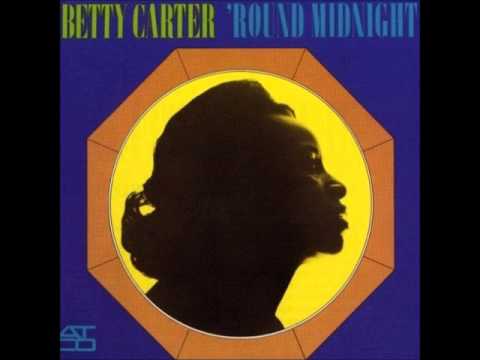
>>54863
I cant get a bite of bread trough my throat without lubricant.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54865
>>54864
that sounds gross!
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54866
>>54857
poor wired
>>54860
a 2D loli gf?
>>54858
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54867
YouTube embed. Click thumbnail to play.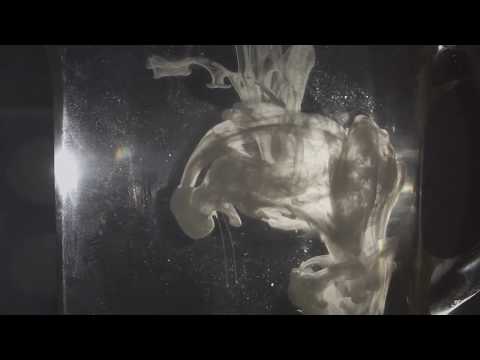
>>54865
What part, the lubricant part or the not getting it trough the throat part?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54868
YouTube embed. Click thumbnail to play.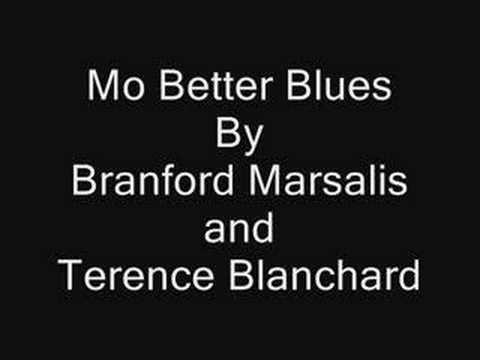
>>54866
Chillin out too some late night jazz atm.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54869
>>54866
Most likely. You must be 2D yourself though, I think.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54870
>>54868
the weekend busy for you, no?
>>54869
we cuddles now?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54871
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54872
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54873
YouTube embed. Click thumbnail to play.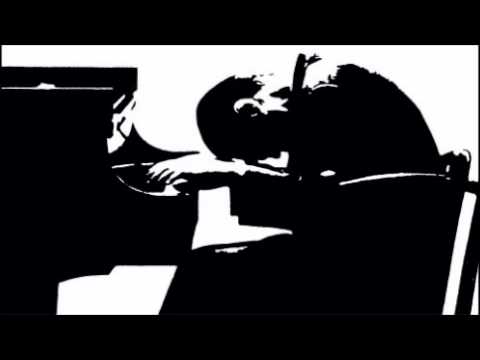
>>54870
Saturday I gots a stupid concert where I need to dress up in tiroller outfits and play crappy skii-party songs.
Sunday evening we celebrating Sinterklaas (Dutch santa kinda deal)
>>54871
They're tasty, and where super cheap. They are a traditional Dutch treat during sinterklaas, which ends the 5th of December. So on the 6th of December all sinterklaas treats go on sale.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54874
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54875
>>54873
oh, it is December, I keep forgetting
you work a government job, won't the sand niggers feel something if you're forced to do that?
>>54874
only if it is okay with you
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54876
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54877
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54878
YouTube embed. Click thumbnail to play.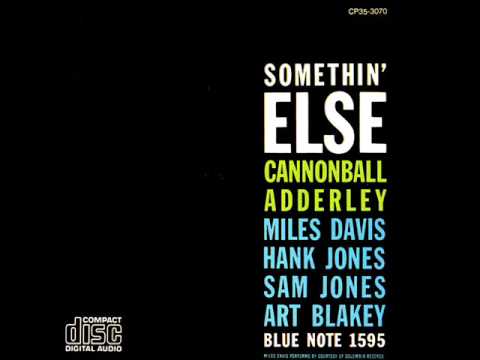
>>54875
Not government owned,been privatised like a decade ago. The neighbourhood I deliver is full of young white families tho, so im not scared of mudslimes
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54879
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54880
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54881
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54882
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54883
>>54877
~
>>54878
the post service in the socialist utopia of Germany is privatized
hmmm… you learn something everyday it seems
at least you don't have to worry about mudslimes killing you for it
>>54879
>>54880
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54884
YouTube embed. Click thumbnail to play.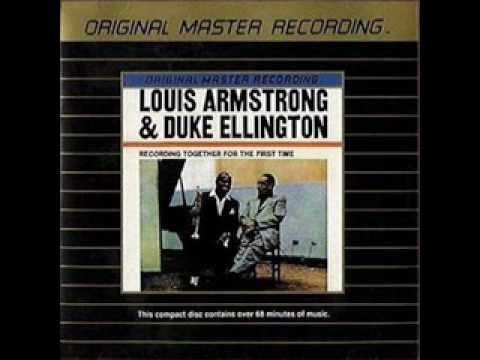
>>54883
>Germany
The Netherlands*, Tyvm
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54885
>>54884
>Netherlands
any Western European country really
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54886
YouTube embed. Click thumbnail to play.
>>54885
Atleast my country isn't leading the front to creating a united communist states of yurope.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54887
>>54886
Netherlands is definitely not the worst
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54888
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54889
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54890
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54891
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54892
YouTube embed. Click thumbnail to play.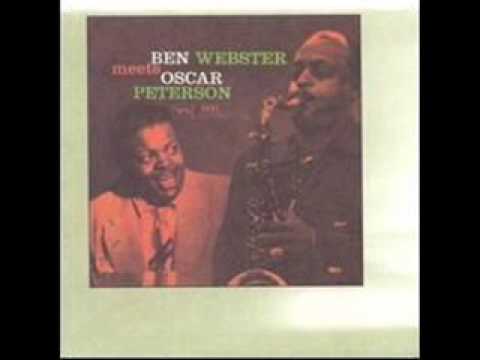
>>54887
Make the Netherlands great again. back to the 17th century. The Golden Age
https://en.wikipedia.org/wiki/Dutch_Golden_Age
>>54888 (checked)
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54893
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54894
>>54879
Also, have a slightly lewd Hotaru.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54895
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54896
YouTube embed. Click thumbnail to play.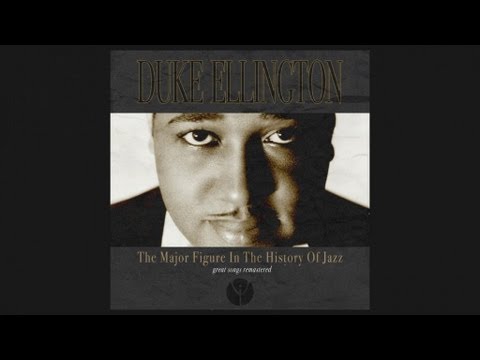
Imma go read up on an adventure module I thinks. Gunna be slow to respond if at all
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54897
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54898
>>54892
you can't go back unless you're willing to sacrifice technological prowess.
and nothing is worth imo
the most you can hope for is gene editing technology, that makes the genetic population within your county super humans, making it not possible not sand niggers or any other economic migrant to compete with your native population as they aren't super.
I propose this plan over automation taking taking care of the sand nigger problem, as it won't take care of female hypergamy, thus your country's population will dwindled to the point of non-existence.
>>54893
~~~*~*
>>54895
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54899
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54900
YouTube embed. Click thumbnail to play.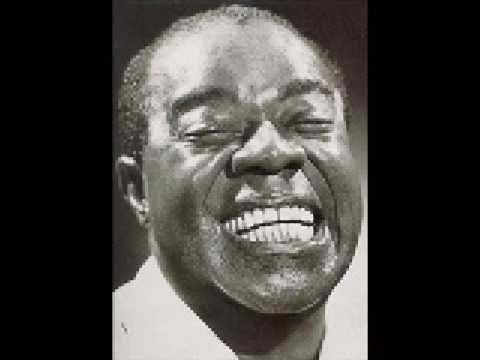
>>54895
>>54898
Just uplaod my brain to a cumputor and let me life forever in the matrix.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54901
>>54899
sorry was picking up brother
>>54900
you can't do that as far as we understand consciousness
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54902
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54903
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54904
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54905
>>54904
just get in my van, okay
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54906
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54907
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54908
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54909
>>54908
wow, that one is nice~
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54910
>>54907
who said anything about lewding?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54911
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54912
>>54910
It just seemed fishy to me.
I AM ALSSSSSSSO A RABBIT
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54913
 | Rolled 18 (1d20) |
>>54910
*Makes a perception check*
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54914
>>54910
>>54913
Ssseemsss, sssussspisssiousss…
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54915
>>54911
he thinks they are his babies
>>54912
I bet you are
>>54913
>>54914
umm.. okay?
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54916
>>54915
>Perception. Your Wisdom (Perception) check lets you spot, hear, or otherwise detect the presence of something. It measures your general awareness of your surroundings and the keenness of your senses. For example, you might try to hear a conversation through a closed door, eavesdrop under an open window, or hear monsters moving stealthily in the forest. Or you might try to spot things that are obscured or easy to miss, whether they are orcs lying in ambush on a road, thugs hiding in the shadows of an alley, or candlelight under a closed secret door.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54917
>>54916
I always cheat in RPGs, so i never lose
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54918
>>54917
Good luck cheating in D&D though, gonna piss of the other people and the GM at the table.
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54919
>>54918
I have no irl friends to play with, so no worries there either
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54920
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54921
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54922
>>54921
I don't understand where that came from X3
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54923
>>54922
I don't understand either
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54924
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54925
>>54924
I going thorough my xeno and captions folder, it got me worked up
I am fine now I think
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54926
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54927
>>54925
Aha, I see.
Well. I should go to bed now. Good night Monsterboy.
And good night/tripfriend/
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54928
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54929
>>54927
good night, mouse
hope you have a good sleep
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.
No.54931
Disclaimer: this post and the subject matter and contents thereof - text, media, or otherwise - do not necessarily reflect the views of the 8kun administration.